Note: Do the topics in sequence: each topic builds on the previous one. Before continuing, make sure you have completed Part 1.
Adding objects (complex variables) to the Watch window
In the first part of the tutorial you have learned to work with simple variables, holding numbers or text. Modern JavaScript coding often relies on objects (complex variables): they are handy because they combine the variables comprising them with functions operating on them.
Launch your JavaScript Editor and open the JavaScript_Debugger_Tutorial_2.htm file. You will find it in the Tutorial folder inside your JavaScript Editor folder.
Take a moment to familiarize yourself with the code. The function Car() constructs the Car object, and the member function toString() constructs its text description using the member variables.
Highlight the MyModel variable (line 41) and click on the Add to Watch button. This opens the Watch window and adds the MyModel variable to the watch list.
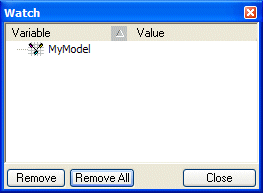
The Watch window automatically updates all the variables being watched whenever the execution is stopped (e.g. by a breakpoint). This allows you to observe the variable at different stages of the program execution. |
Tip: Resize the Watch window to the size that suits you best: it remembers its size and position. |
Highlight BMW.year (line 37) and click on the Add to Watch button.
Note that the values for the MyModel and BMW.year variables are not displayed yet. They will be updated when we start the debugging session and reach the first breakpoint.
Click on the Start Debugging button. The browser with the appears with the JavaScript_Debugger_Tutorial_2.htm file.
Switch back to the JavaScript Editor and set the breakpoint on line 47:

Switch back to the browser and click on the BMW button.
The breakpoint is reached, JavaScript Editor springs into view and displays where the execution is stopped. Note that the Watch window now displays the values of the variables being watched.
Double-click on the MyCar variable (line 46) to highlight it and click on the Add to Watch button.
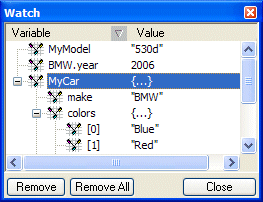
The MyCar variable is an object. Therefore, the Watch window lists all of its member variables. Because MyCar.colors is an object itself (an array), all the members of the array are listed.
Summary: Use the Watch window to follow the state of the variables being watched at different stages of the program execution. |
Fast way to find out the value of a variable
You will often need to know the value variables are holding at some point of the program execution. If that is all you want, there is no need to add them to the Watch or use the Variables or Stack Frames windows.
The fastest way to find out the value a variable is holding is to highlight it and click on the Inspect Variable button. |
Highlight the MyModel2 variable (line 42), and click on the Inspect Variable button.
Highlight the BMW.colors[2] variable (line 34), and click on the Inspect Variable button.
The values of MyModel2 and BMW.colors[2] are shown in the Output tab of the Project pane. To terminate the debugging session:
Click on the Continue button, and
Click on the Stop Debugging button.
How to debug the code executing as the page is loading
Up until now in all the examples the debugging session starts after the page has been loaded. Most of the time this is exactly what you want. But what if you want to debug the code executing as the page is loading?
Unlike the competition, JavaScript Editor gives you a simple and amazingly easy way to do it:
With the JavaScript_Debugger_Tutorial_2.htm file still open in the Editor, click on the Start Debugging button. The browser running your document appears.
Select Build / Break from the menu.
Switch to the browser and click on the Refresh button to reload the current document.
JavaScript Editor springs into view. Notice that the execution stopped at the first line of script:

Keep in mind that because the document has been reloaded you cannot set or use the breakpoints without disconnecting the debugger and reconnecting it.
However, you can now step through the code using Step Into, Step Over and Step Out, add variables to the Watch, inspect them, etc.
To terminate the debugging session:
Click on the Continue button, and
Click on the Stop Debugging button.
Connecting the debugger to the running instance of Internet Explorer
To debug your code instantly all you have to do is a) load the page in JavaScript Editor and b) click the Start Debugging button.
In addition and as an advanced feature, JavaScript Editor allows you to connect its debugger to any instance of Internet Explorer. This can be the Internet Explorer itself, or any application embedding the Internet Explorer object. JavaScript debugger built into JavaScript Editor cannot connect to Mozilla-based browsers (e.g. Firefox).
With the JavaScript_Debugger_Tutorial_2.htm file still open in the Editor, select View / Launch - View in Browser from the menu. The browser running your document appears.
Select Build / Connect Debugger from the menu.
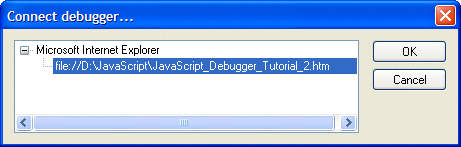
The dialog lists all the instances of Internet Explorer and the documents they are running.
Select JavaScript_Debugger_Tutorial_2.htm and click OK.
JavaScript debugger is now connected. You can set breakpoints, inspect variables, and use all the debugging commands.
To terminate the debugging session:
Click on the Continue button (if the execution has been stopped)
Click on the Stop Debugging button, and
Switch over to the browser and close it down.
Congratulations on completing the JavaScript debugger tutorial! The know-how you acquired guarantees speedy debugging of your web applications.
Happy coding!
Dr Alex and the C Point team
P.S. Have you checked recently the download page for JavaScript Editor components? Perhaps a new component has been added to make your coding experience with JavaScript Editor even more pleasurable. |