No programming language to-date has yet eliminated programming mistakes. You can swiftly locate many types of errors using JavaScript Editor, but logical errors are more difficult to spot.
Below are some common mistakes to avoid:
Comparisons
Unlike strongly-typed languages (C#, C++...) in JavaScript you do not have to specify the storage type when declaring your variables. With some comparisons, this simplicity may pose a problem. Consider the following code fragment:
currentYear = "2006";
taxRate = 25;
// ...
if(currentYear == 2006) taxRate = 25;
else taxRate = 35;
document.write(taxRate);
|
To run the example, paste it into JavaScript Editor, and click the Execute button.
What happened? The test "2006" == 2006 evaluates to true, even though one is a string and the other one is a number. In JavaScript, "2005" and 2005 are the same, but not identical.
You can also test if two objects are identical:
currentYear = "2006";
taxRate = 25;
// ...
if(currentYear === 2006) taxRate = 25; // Note: === instead of ==
else taxRate = 35;
document.write(taxRate);
|
To run the example, paste it into JavaScript Editor, and click the Execute button.
What happened this time? "2006" and 2006 are not identical, so the taxRate is set to to 35.
Order of execution
In JavaScript (and other languages) operators are evaluated in the order of precedence. If the operators are of equal importance, than left-to-right order is used. To check the order of precedence for JavaScript operators, click here. Example:
result = 10-7%3;
document.write(result);
|
To run the example, paste it into JavaScript Editor, and click the Execute button.
The modulo divider operator % takes precedence over the subtraction - operator. Therefore, 7%3 is evaluated first (the result is 1), followed by 10-1.
When you place JavaScript into your web pages, the part between the <head> </head> tags is evaluated first. Because of that, never place any code in the <head> section that depends on the code in the <body> section.
When iterating through object properties using the for...in statement, you cannot rely on a particular order of properties.
Syntax errors
Examples of syntax errors are mistyping the name of the variable or forgetting to close a string, mismatched parentheses or braces, etc. When you use JavaScript in your web pages, the browser will not report an error unless it tries to execute that part of the script.
Some parts of the script might be rarely executed, with the errors remaining hidden for days, months or even years. |
Having hidden syntax errors is never the problem with JavaScript Editor: finding syntax errors is easy:
Click on the Syntax Check button (or press Ctrl+F8)
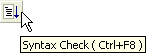
If you made an error, JavaScript Editor will locate it in no time, highlight it and explain it for a speedy correction:

Important: Syntax Check is not limited to JavaScript used in the context of web pages. It works when you use JavaScript for other purposes as well, for example for file processing on your computer or your intranet.
This gives you an unprecedented freedom to use JavaScript not only for your web pages, but for solving many common computing problems. |
To find out more, please see the Syntax check section of the JavaScript Editor reference.
Object referencing
Improper use of the this keyword: the this keyword is used inside functions, but it points to the object, not the function. You cannot use it to point to the function itself.
Referencing an object before it has been loaded: this typically happens in your JavaScript code in the <head> section tries to manipulate objects defined in the <body> section.
Using reserved words as variable names
Although using one of the reserved words to name a variable is possible in JavaScript, you should avoid doing it. After the variable has been declared, using it with the reserved word in the same scope can produce all kinds of problems.
For a list of JavaScript keywords, click here.
Compatibility problems
Web browsers have differences in their implementation and support of JavaScript. In some cases, your code may have to detect the browser using it, and execute the code meant only for a particular browser.
To check the name of the browser and its version, open JavaScript Editor's Solutions pane, Misc/BrowserCheck.js file.
Next
|